diff --git a/2025/sketch_2025_01_04/sketch_2025_01_04.png b/2025/sketch_2025_01_04/sketch_2025_01_04.png
new file mode 100644
index 00000000..7ef42243
Binary files /dev/null and b/2025/sketch_2025_01_04/sketch_2025_01_04.png differ
diff --git a/2025/sketch_2025_01_04/sketch_2025_01_04.py b/2025/sketch_2025_01_04/sketch_2025_01_04.py
new file mode 100644
index 00000000..89414cc8
--- /dev/null
+++ b/2025/sketch_2025_01_04/sketch_2025_01_04.py
@@ -0,0 +1,44 @@
+import py5
+from py5 import sin, cos, PI
+
+def setup():
+ py5.size(800, 800)
+ py5.translate(400, 400)
+ py5.background(24)
+ py5.stroke(0)
+ py5.fill(0)
+ wave(390)
+ py5.save('out.png')
+
+def wave(r):
+ vs = circle_arc_pts(0, 0, r, 0, py5.PI)
+ vs += reversed(circle_arc_pts(-r/2, 0, r/2, 0, py5.PI))
+ vs += reversed(circle_arc_pts(r/2, 0, r/2, 0, py5.PI))
+ with py5.begin_shape():
+ py5.vertices(vs)
+ if r > 20:
+ with py5.push_matrix():
+ py5.translate(-r/2, 0)
+ py5.rotate(py5.PI/3)
+ wave(r/2)
+ py5.rotate(-py5.PI/3)
+ py5.translate(r, 0)
+ py5.rotate(py5.PI/2)
+ wave(r/2)
+
+def circle_arc_pts(x, y, radius, start, sweep):
+ return arc_pts(x, y, radius * 2, radius * 2, start, start + sweep)
+
+def arc_pts(cx, cy, w, h, start_angle, end_angle, num_points=24):
+ sweep_angle = end_angle - start_angle
+ pts_list = []
+ step_angle = float(sweep_angle) / num_points
+ va = start_angle
+ side = 1 if sweep_angle > 0 else -1
+ while va * side < end_angle * side or abs(va - end_angle) < 0.0001:
+ pts_list.append((cx + cos(va) * w / 2.0,
+ cy + sin(va) * h / 2.0))
+ va += step_angle
+ return pts_list
+
+py5.run_sketch()
\ No newline at end of file
diff --git a/docs/README.md b/docs/README.md
index 72d634ba..f83d94e0 100644
--- a/docs/README.md
+++ b/docs/README.md
@@ -21,6 +21,16 @@ If you appreciate what I have been doing, you may also support my artistic work,
2025 \| [2024](2024.md) \| [2023](2023.md) \| [2022](2022.md) \| [2021](2021.md) \| [2020](2020.md) \| [2019](2019.md) \| [2018](2018.md)
+---
+
+### sketch_2025_01_04
+
+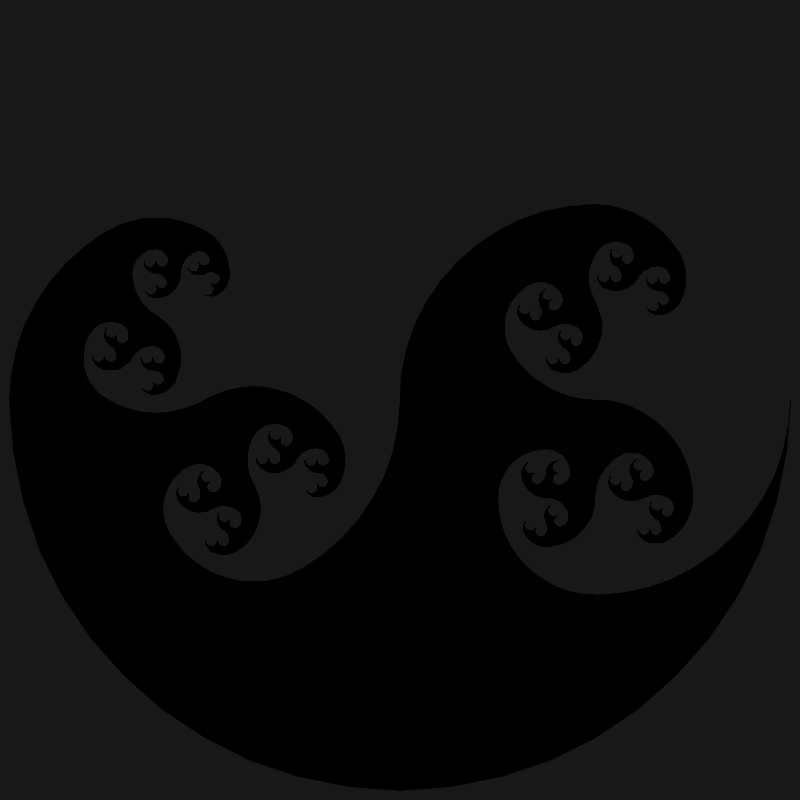
+
+[sketch_2025_01_04](https://github.com/villares/sketch-a-day/tree/main/2025/sketch_2025_01_04) [[py5](https://py5coding.org/)]
+
+
+
---
### sketch_2025_01_03