diff --git a/2025/sketch_2025_01_06/out024.png b/2025/sketch_2025_01_06/out024.png
new file mode 100644
index 00000000..728363a6
Binary files /dev/null and b/2025/sketch_2025_01_06/out024.png differ
diff --git a/2025/sketch_2025_01_06/out036.png b/2025/sketch_2025_01_06/out036.png
new file mode 100644
index 00000000..45d67098
Binary files /dev/null and b/2025/sketch_2025_01_06/out036.png differ
diff --git a/2025/sketch_2025_01_06/out041.png b/2025/sketch_2025_01_06/out041.png
new file mode 100644
index 00000000..c80da986
Binary files /dev/null and b/2025/sketch_2025_01_06/out041.png differ
diff --git a/2025/sketch_2025_01_06/out046.png b/2025/sketch_2025_01_06/out046.png
new file mode 100644
index 00000000..7a20e04b
Binary files /dev/null and b/2025/sketch_2025_01_06/out046.png differ
diff --git a/2025/sketch_2025_01_06/sketch_2025_01_06.png b/2025/sketch_2025_01_06/sketch_2025_01_06.png
new file mode 100644
index 00000000..f4ae4d17
Binary files /dev/null and b/2025/sketch_2025_01_06/sketch_2025_01_06.png differ
diff --git a/2025/sketch_2025_01_06/sketch_2025_01_06.py b/2025/sketch_2025_01_06/sketch_2025_01_06.py
new file mode 100644
index 00000000..6771cee2
--- /dev/null
+++ b/2025/sketch_2025_01_06/sketch_2025_01_06.py
@@ -0,0 +1,73 @@
+# Delaunay play
+from itertools import product
+
+import py5
+from scipy.spatial import Delaunay
+import numpy as np
+
+num_points = 200
+seed = 0
+
+def setup():
+ py5.size(600, 600, py5.P3D)
+ py5.color_mode(py5.HSB)
+ new_points()
+
+def new_points():
+ global seed_points, tris
+ W = 60
+ R = 20
+ py5.random_seed(seed)
+ seed_points = []
+ for i in range(2):
+ seed_points.extend((x + (i - 1) * py5.random(-R, R),
+ y + i * py5.random(-R, R),
+ i * R
+ ) for x, y
+ in product(range(0, py5.width + W, W),
+ range(0, py5.height+ W, W)))
+ pts = np.array(seed_points)
+ tri = Delaunay(seed_points)
+ tris = pts[tri.simplices]
+
+def draw():
+ py5.random_seed(seed)
+ py5.background(0)
+ py5.translate(-50, -50, 50)
+ py5.rotate_x(py5.radians(30))
+ #py5.scale(500 / 600)
+ for vs in tris:
+ shp = py5.create_shape()
+ with shp.begin_closed_shape():
+ shp.vertices(vs)
+ shp.set_fills(noise_colors(vs))
+ py5.shape(shp)
+# f = py5.frame_count
+# if f < 361 and f % 5 == 0:
+# py5.save_frame('###.png')
+
+
+
+def noise_colors(vs):
+ step = py5.TAU / len(vs)
+ colors = []
+ for i, (xo, yo, zo) in enumerate(vs):
+ a = i * step + py5.radians(py5.frame_count)
+ x = py5.cos(a) + xo / 10
+ y = py5.sin(a) + yo / 10
+ h = 255 * (1 + py5.os_noise(x, y, 100)) / 2
+ s = 255 * (1 + py5.os_noise(x, y, 1000)) / 2
+ b = 255 #* (1 + py5.os_noise(x, y, 10000)) / 2
+ colors.append(py5.color(h, s, b))
+ return colors
+
+def key_pressed():
+ global seed
+ if py5.key == ' ':
+ seed += 1
+ new_points()
+ elif str(py5.key).lower() == 's':
+ py5.save_frame('out###.png')
+
+
+py5.run_sketch(block=False)
\ No newline at end of file
diff --git a/docs/README.md b/docs/README.md
index c02da3f1..9d846187 100644
--- a/docs/README.md
+++ b/docs/README.md
@@ -21,6 +21,16 @@ If you appreciate what I have been doing, you may also support my artistic work,
2025 \| [2024](2024.md) \| [2023](2023.md) \| [2022](2022.md) \| [2021](2021.md) \| [2020](2020.md) \| [2019](2019.md) \| [2018](2018.md)
+---
+
+### sketch_2025_01_06
+
+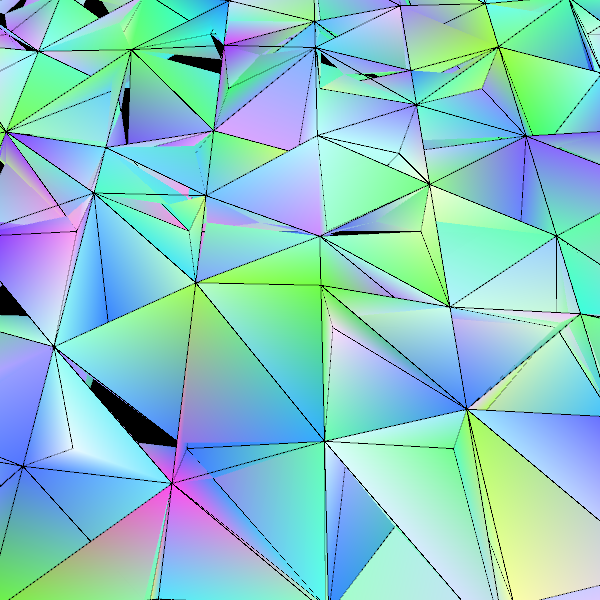
+
+[sketch_2025_01_06](https://github.com/villares/sketch-a-day/tree/main/2025/sketch_2025_01_06) [[py5](https://py5coding.org/)]
+
+
+
---
### sketch_2025_01_05