diff --git a/HandyUI/Models/FileManagerModel.cs b/HandyUI/Models/FileManagerModel.cs
new file mode 100644
index 0000000..8785434
--- /dev/null
+++ b/HandyUI/Models/FileManagerModel.cs
@@ -0,0 +1,13 @@
+using System.Windows.Media;
+
+namespace HandyUI.Models
+{
+ public class FileManagerModel
+ {
+ public DrawingImage Image { get; set; }
+ public string Name { get; set; }
+ public string FileItem { get; set; }
+ public string LastModified { get; set; }
+ public string FileSize { get; set; }
+ }
+}
diff --git a/HandyUI/Resources/Geometries.xaml b/HandyUI/Resources/Geometries.xaml
index e4e339c..d377749 100644
--- a/HandyUI/Resources/Geometries.xaml
+++ b/HandyUI/Resources/Geometries.xaml
@@ -1530,7 +1530,6 @@
-
@@ -1561,7 +1560,6 @@
-
@@ -1587,6 +1585,420 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
M3 2C1.895 2 1 2.895 1 4L1 16C1 17.105 1.895 18 3 18L10 18L10 20L8 20L8 22L16 22L16 20L14 20L14 18L21 18C22.105 18 23 17.105 23 16L23 4C23 2.895 22.105 2 21 2L3 2 z M 3 4L21 4L21 16L3 16L3 4 z
M18,2H8C6.897,2,6,2.897,6,4v5.586L4.586,11C4.214,11.372,4,11.888,4,12.414V20c0,1.103,0.897,2,2,2h12c1.103,0,2-0.897,2-2 V4C20,2.897,19.103,2,18,2z M10,7H8V4h2V7z M14,7h-2V4h2V7z M18,7h-2V4h2V7z
M8.5 4 A 3.5 3.5 0 0 0 5 7.5 A 3.5 3.5 0 0 0 8.5 11 A 3.5 3.5 0 0 0 12 7.5 A 3.5 3.5 0 0 0 8.5 4 z M 17 7 A 3 3 0 0 0 14 10 A 3 3 0 0 0 17 13 A 3 3 0 0 0 20 10 A 3 3 0 0 0 17 7 z M 8.5 13C5.69 13 3.2395313 14.140313 2.0195312 14.820312C1.3895312 15.180312 1 15.840312 1 16.570312L1 20L9 20L9 17.130859C9 15.710859 9.7790625 14.379922 11.039062 13.669922C11.179062 13.589922 11.339766 13.509922 11.509766 13.419922C10.589766 13.169922 9.57 13 8.5 13 z M 17 14C14.858 14 12.963437 14.886063 12.023438 15.414062C11.392437 15.770062 11 16.427859 11 17.130859L11 20L23 20L23 17.130859C23 16.427859 22.606609 15.770063 21.974609 15.414062C21.035609 14.886062 19.142 14 17 14 z
diff --git a/HandyUI/ViewModels/FileManagerViewModel.cs b/HandyUI/ViewModels/FileManagerViewModel.cs
index 7d55103..5651ba7 100644
--- a/HandyUI/ViewModels/FileManagerViewModel.cs
+++ b/HandyUI/ViewModels/FileManagerViewModel.cs
@@ -1,12 +1,31 @@
-using Prism.Mvvm;
+using HandyUI.Models;
+using Prism.Mvvm;
+using System;
+using System.Collections.ObjectModel;
+using System.Windows;
+using System.Windows.Media;
namespace HandyUI.ViewModels
{
public class FileManagerViewModel : BindableBase
{
- public FileManagerViewModel()
+ ResourceDictionary dict = Application.LoadComponent(new Uri("HandyUI;component/Resources/Geometries.xaml", UriKind.RelativeOrAbsolute)) as ResourceDictionary;
+
+ private ObservableCollection _data = new ObservableCollection();
+ public ObservableCollection data
{
+ get { return _data; }
+ set { SetProperty(ref _data, value); }
+ }
+ public FileManagerViewModel()
+ {
+ data.Add(new FileManagerModel { Image = (DrawingImage)dict["folder"], Name = "Collection 1", FileItem = "21 Items", LastModified = "Jan 3, 2019", FileSize = "15 Gb" });
+ data.Add(new FileManagerModel { Image = (DrawingImage)dict["folder"], Name = "Collection 2", FileItem = "40 Items", LastModified = "Jan 10, 2020", FileSize = "45 Gb" });
+ data.Add(new FileManagerModel { Image = (DrawingImage)dict["folder"], Name = "Collection 3", FileItem = "90 Items", LastModified = "Mar 10, 2020", FileSize = "82 Gb" });
+ data.Add(new FileManagerModel { Image = (DrawingImage)dict["video"], Name = "File Manager UI Part-1 by Jd's Code Lab", FileItem = "1 Item", LastModified = "Jun 14, 2020", FileSize = "1 Gb" });
+ data.Add(new FileManagerModel { Image = (DrawingImage)dict["video"], Name = "File Manager UI Part-2 by Jd's Code Lab", FileItem = "1 Item", LastModified = "Jun 17, 2020", FileSize = "4 Gb" });
+ data.Add(new FileManagerModel { Image = (DrawingImage)dict["music"], Name = "Some music track", FileItem = "1 Items", LastModified = "March 19, 2019", FileSize = "5 Mb" });
}
}
}
diff --git a/HandyUI/Views/FileManager.xaml b/HandyUI/Views/FileManager.xaml
index 7ab71e6..8c423f4 100644
--- a/HandyUI/Views/FileManager.xaml
+++ b/HandyUI/Views/FileManager.xaml
@@ -99,16 +99,157 @@
-
-
-
-
-
+
-
-
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/README.md b/README.md
index f20d788..e6e1a78 100644
--- a/README.md
+++ b/README.md
@@ -17,6 +17,8 @@ We use [Dribbble](https://dribbble.com/) designs
## Do you Want to contribute?
Why did you wait? Fix one of the above issues or create a new user interface.
+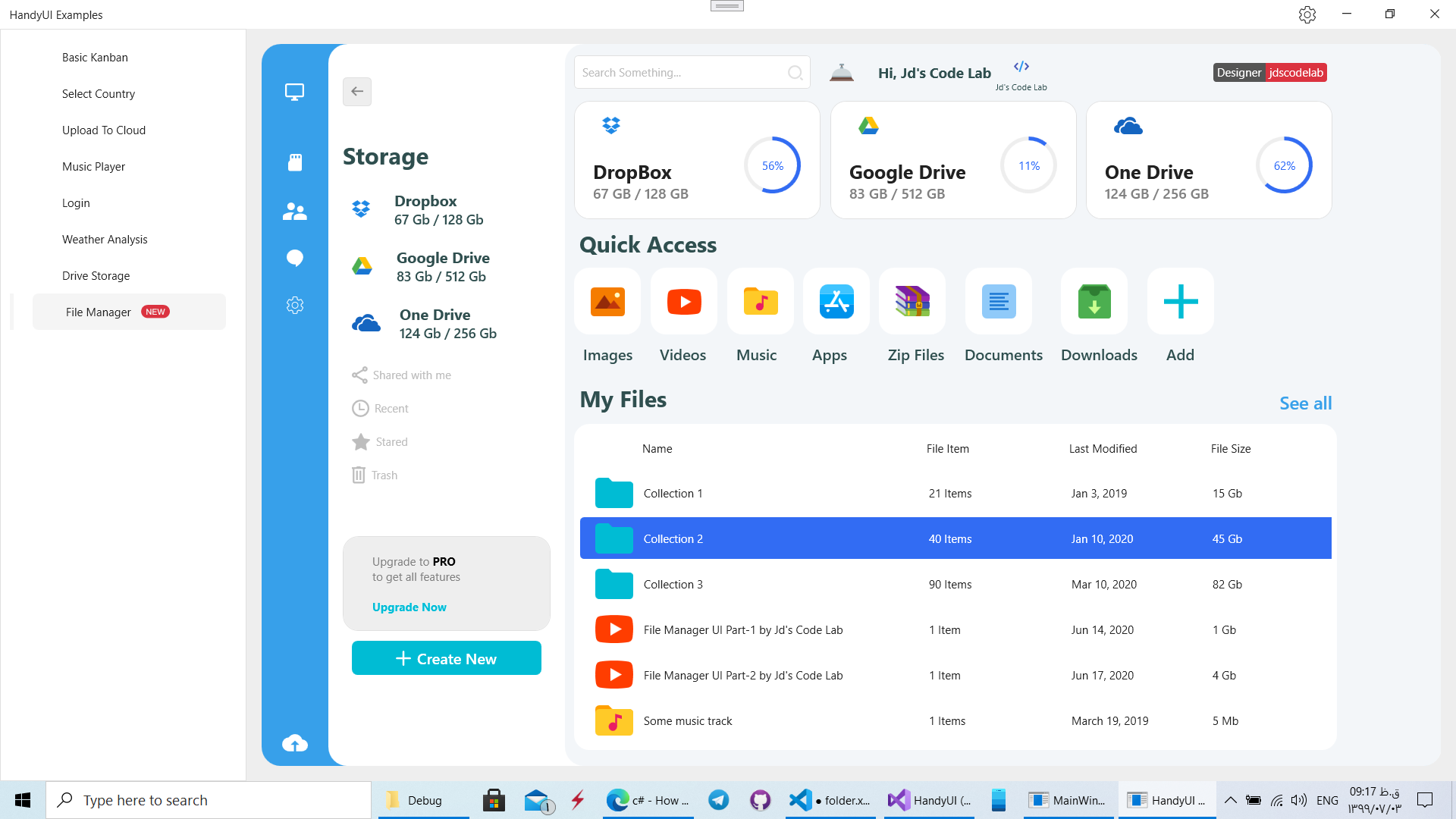
+
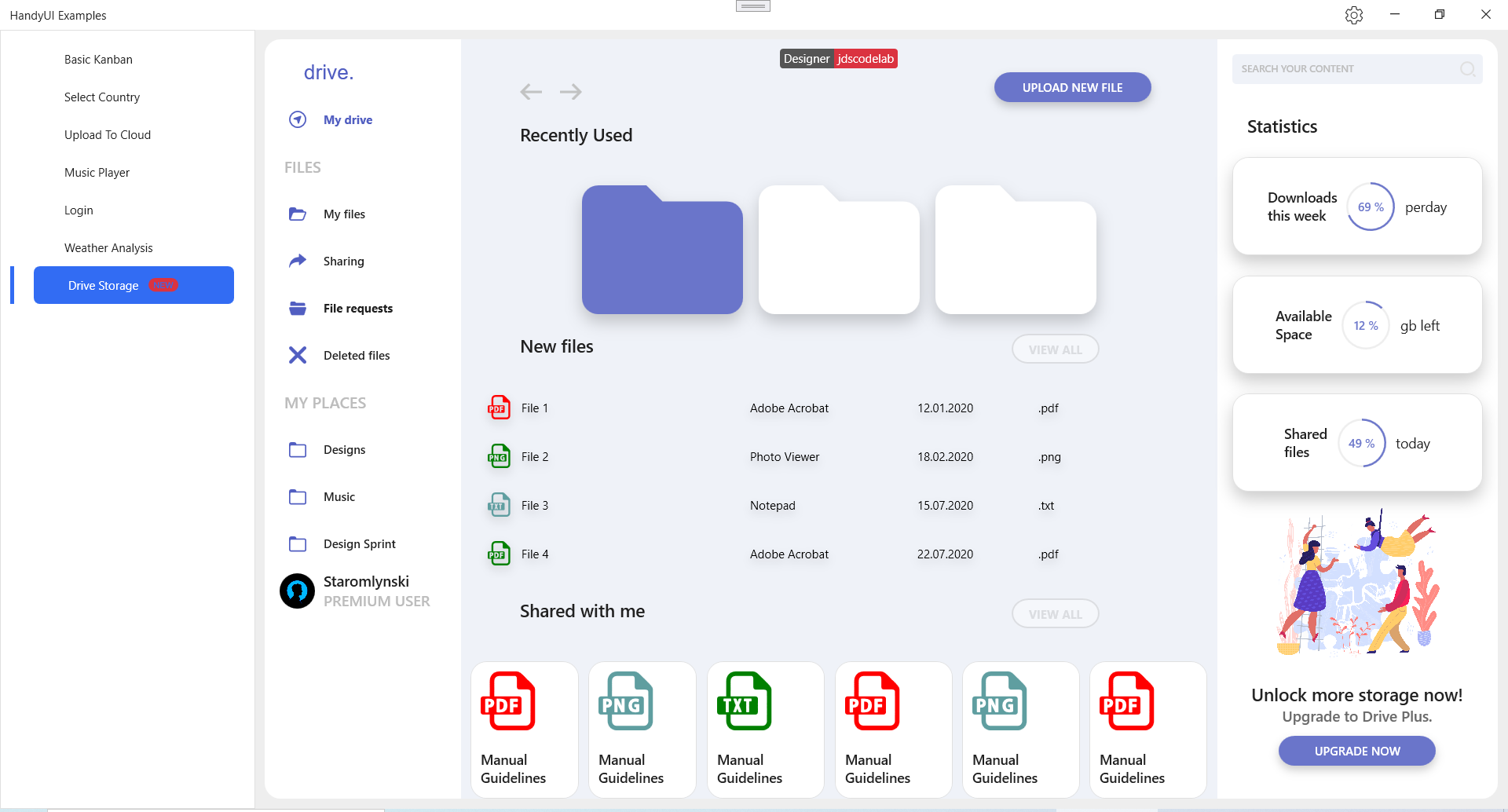
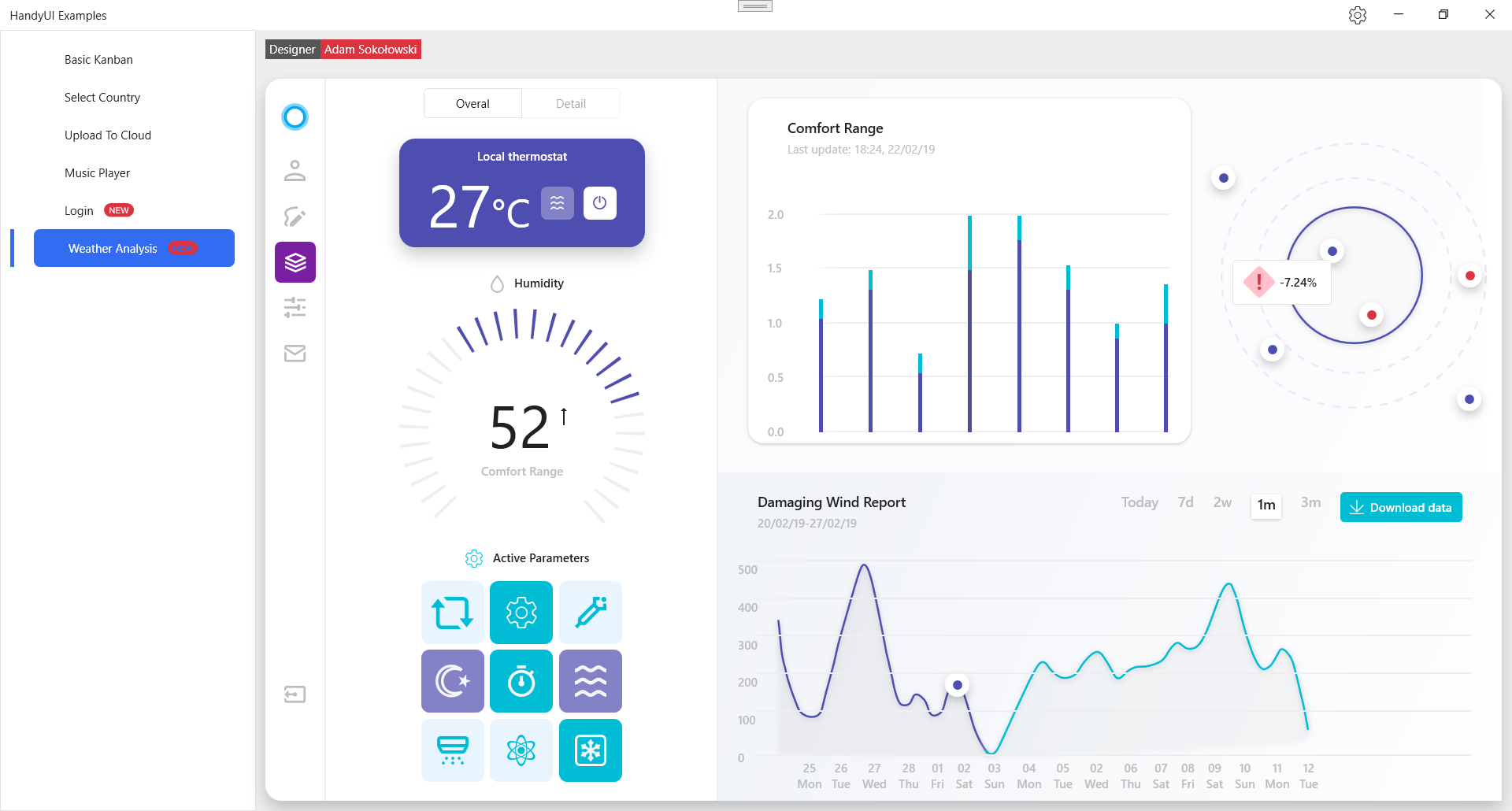