diff --git a/README.md b/README.md
index 1b36ac90..e94d3c67 100644
--- a/README.md
+++ b/README.md
@@ -517,6 +517,9 @@ Users can build their own custom filters which can be used from the front-end us
import pandas as pd
import dtale
import dtale.predefined_filters as predefined_filters
+import dtale.global_state as global_state
+
+global_state.set_app_settings(dict(open_predefined_filters_on_startup=True))
predefined_filters.set_filters([
{
@@ -525,6 +528,8 @@ predefined_filters.set_filters([
"description": "Filter A with B greater than 2",
"handler": lambda df, val: df[(df["A"] == val) & (df["B"] > 2)],
"input_type": "input",
+ "default": 1,
+ "active": False,
},
{
"name": "A and (B % 2) == 0",
@@ -532,6 +537,8 @@ predefined_filters.set_filters([
"description": "Filter A with B mod 2 equals zero (is even)",
"handler": lambda df, val: df[(df["A"] == val) & (df["B"] % 2 == 0)],
"input_type": "select",
+ "default": 1,
+ "active": False,
},
{
"name": "A in values and (B % 2) == 0",
@@ -539,6 +546,8 @@ predefined_filters.set_filters([
"description": "A is within a group of values and B mod 2 equals zero (is even)",
"handler": lambda df, val: df[df["A"].isin(val) & (df["B"] % 2 == 0)],
"input_type": "multiselect",
+ "default": [1],
+ "active": True,
}
])
@@ -555,7 +564,7 @@ This code illustrates the types of inputs you can have on the front end:
* __multiselect__: same as "select" but it will allow you to choose multiple values (handy if you want to perform an `isin` operation in your filter)
Here is a demo of the functionality:
-[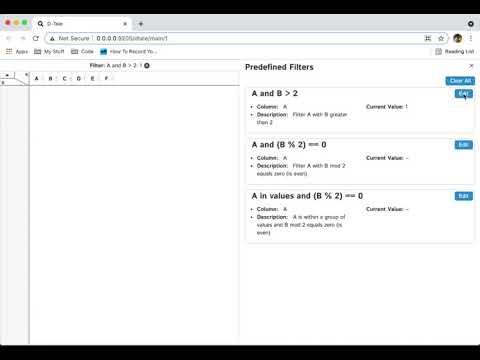](http://www.youtube.com/watch?v=5E_dkVJizcY "Predefined Filters")
+[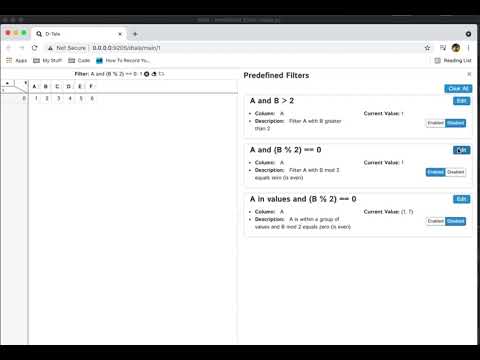](http://www.youtube.com/watch?v=8mryVxpxjM4 "Predefined Filters")
If there are any new types of inputs you would like available please don't hesitate to submit a request on the "Issues" page of the repo.
diff --git a/docs/CONFIGURATION.md b/docs/CONFIGURATION.md
index 52e8f759..6c1fbc41 100644
--- a/docs/CONFIGURATION.md
+++ b/docs/CONFIGURATION.md
@@ -17,6 +17,13 @@ hide_shutdown = False
pin_menu = False
language = en
max_column_width = 100 # the default value is None
+main_title = My App # only use this if you don't want to see the D-Tale logo
+main_title_font = Arial # this font is applied to your custom title
+query_engine = python
+
+[charts] # this controls how many points can be contained within scatter & 3D charts
+scatter_points = 15000
+3d_points = 4000
[auth]
active = True
@@ -34,6 +41,11 @@ allow_cell_edits = True
inplace = False
drop_index = False
app_root = additional_path
+precision = 6 # how many digits to show on floats
+show_columns = a,b
+hide_columns = c
+column_formats = {"a": {"fmt": {"html": true}}}
+sort = a|ASC,b|DESC
```
Some notes on these properties:
diff --git a/dtale/predefined_filters.py b/dtale/predefined_filters.py
index f7faf238..5afdd4e7 100644
--- a/dtale/predefined_filters.py
+++ b/dtale/predefined_filters.py
@@ -84,7 +84,8 @@ def init_filters():
global PREDEFINED_FILTERS
return {
- f.name: dict(value=f.default, active=f.get("active", True))
- for f in PREDEFINED_FILTERS
+ f.name: dict(value=f.default, active=f.active)
if f.default is not None
+ else dict(active=f.active)
+ for f in PREDEFINED_FILTERS
}
diff --git a/static/dtale/gridUtils.jsx b/static/dtale/gridUtils.jsx
index d2eb20e2..dd96ea4d 100644
--- a/static/dtale/gridUtils.jsx
+++ b/static/dtale/gridUtils.jsx
@@ -218,7 +218,16 @@ export const buildState = props => ({
export const noHidden = columns => !_.some(columns, { visible: false });
-export const filterPredefined = filters => _.pickBy(filters, value => value.active && value.value);
+export const predefinedHasValue = value => {
+ if (value?.value === undefined) {
+ return false;
+ }
+ if (Array.isArray(value.value) && _.isEmpty(value.value)) {
+ return false;
+ }
+ return true;
+};
+export const filterPredefined = filters => _.pickBy(filters, value => value.active && predefinedHasValue(value));
export const noFilters = ({ query, columnFilters, outlierFilters, predefinedFilters }) =>
_.isEmpty(query) &&
_.isEmpty(columnFilters) &&
diff --git a/static/dtale/side/predefined_filters/FilterInput.jsx b/static/dtale/side/predefined_filters/FilterInput.jsx
index 0467ace0..e76b5642 100644
--- a/static/dtale/side/predefined_filters/FilterInput.jsx
+++ b/static/dtale/side/predefined_filters/FilterInput.jsx
@@ -205,7 +205,7 @@ class FilterInput extends React.Component {
{!edit && (
<>
{t("predefined:Current Value")}:
- {value?.value ? predefinedFilterStr([filter], name, value.value) : "--"}
+ {gu.predefinedHasValue(value) ? predefinedFilterStr([filter], name, value.value) : "--"}
>
)}
{this.renderEdited()}