Writing Lighthouse data to a crazyflie #321
Replies: 3 comments 1 reply
-
For your convenience, I have copy and pasted the exact persist file I am trying to run as it appears when I am running it: #!/usr/bin/env python3
# ,---------, ____ _ __
# | ,-^-, | / __ )(_) /_______________ _____ ___
# | ( O ) | / __ / / __/ ___/ ___/ __ `/_ / / _ \
# | / ,--´ | / /_/ / / /_/ /__/ / / /_/ / / /_/ __/
# +------` /_____/_/\__/\___/_/ \__,_/ /___/\___/
#
# Crazyflie control firmware
#
# Copyright (C) 2020 Bitcraze AB
#
# This program is free software: you can redistribute it and/or modify
# it under the terms of the GNU General Public License as published by
# the Free Software Foundation, in version 3.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with this program. If not, see <http://www.gnu.org/licenses/>.
#
#
# Persist geometry and calibration data in the Crazyflie storage.
#
# This script uploads geometry and calibration data to a crazyflie and
# writes the data to persistent memory to make it available after
# re-boot.
#
# This script is a temporary solution until there is support
# in the client.
import logging
from threading import Event
import cflib.crtp # noqa
from cflib.crazyflie import Crazyflie
from cflib.crazyflie.mem import LighthouseBsCalibration
from cflib.crazyflie.mem import LighthouseBsGeometry
from cflib.crazyflie.syncCrazyflie import SyncCrazyflie
from cflib.localization import LighthouseConfigWriter
uri = 'radio://0/80/2M'
class WriteMem:
def __init__(self, uri, geos, calibs):
self._event = Event()
with SyncCrazyflie(uri, cf=Crazyflie(rw_cache='./cache')) as scf:
writer = LighthouseConfigWriter(scf.cf, nr_of_base_stations=2)
writer.write_and_store_config(self._data_written, geos=geos, calibs=calibs)
self._event.wait()
def _data_written(self, sucess):
print('Data written')
self._event.set()
geo0 = LighthouseBsGeometry()
geo0.origin = [-1.01977998,-0.19424433, 1.97086964]
geo0.rotation_matrix = [[ 0.66385385,-0.26347329, 0.6999142 ], [0.18206993,0.96466617,0.19044612], [-0.72536102, 0.00100494, 0.68836792], ]
geo0.valid = True
geo1 = LighthouseBsGeometry()
geo1.origin = [0, 0, 0]
geo1.rotation_matrix = [[1, 0, 0], [0, 1, 0], [0, 0, 1]]
geo1.valid = False
calib0 = LighthouseBsCalibration()
calib0.sweeps[0].tilt = -0.049844
calib0.sweeps[0].phase = 0.000000
calib0.sweeps[0].curve = -0.477082
calib0.sweeps[0].gibphase = 1.870844
calib0.sweeps[0].gibmag = -0.006574
calib0.sweeps[0].ogeephase = 1.916343
calib0.sweeps[0].ogeemag = 0.110889
calib0.sweeps[1].tilt = 0.048504
calib0.sweeps[1].phase = 0.004812
calib0.sweeps[1].curve = 0.545917
calib0.sweeps[1].gibphase = 2.412284
calib0.sweeps[1].gibmag = -0.006277
calib0.sweeps[1].ogeephase = 3.063375
calib0.sweeps[1].ogeemag = 0.202656
calib0.uid = 0x2C0DCDCD
calib0.valid = True
calib1 = LighthouseBsCalibration()
calib1.sweeps[0].tilt = -0.048398
calib1.sweeps[0].phase = 0.000000
calib1.sweeps[0].curve = 0.155712
calib1.sweeps[0].gibphase = 1.315732
calib1.sweeps[0].gibmag = -0.002849
calib1.sweeps[0].ogeephase = 1.291309
calib1.sweeps[0].ogeemag = -0.108465
calib1.sweeps[1].tilt = 0.047573
calib1.sweeps[1].phase = -0.005821
calib1.sweeps[1].curve = 0.246296
calib1.sweeps[1].gibphase = 1.344470
calib1.sweeps[1].gibmag = -0.005334
calib1.sweeps[1].ogeephase = 1.408694
calib1.sweeps[1].ogeemag = 0.039339
calib1.uid = 0xE72CEE73
calib1.valid = True
logging.basicConfig(level=logging.ERROR)
cflib.crtp.init_drivers(enable_debug_driver=False)
WriteMem(uri,
{
0: geo0,
1: geo1,
},
{
0: calib0,
1: calib1,
}) |
Beta Was this translation helpful? Give feedback.
-
Hi! The error you are getting, unexpected nr of memory found, suggest that the lighthouse deck is not connected when you are trying to write the geometry data. The function the writes the data is using the deck driver to write the data to the Crazyflie storage so it requires the deck to be installed and detected. By the way, you should not have to enable anything in the Makefile anymore to enable lighthouse support. The setting_up.md documentation you are linking to is actually quite old (from the 2021.01 release), how did you find this documentation? The website documentation should be up to date (ie. if it is not, it is a bug). |
Beta Was this translation helpful? Give feedback.
-
Just adding some more information: In general, acquiring calibration data through the lightsweeps usually works fine and setting it manually should not be needed. |
Beta Was this translation helpful? Give feedback.
-
Hello everyone,
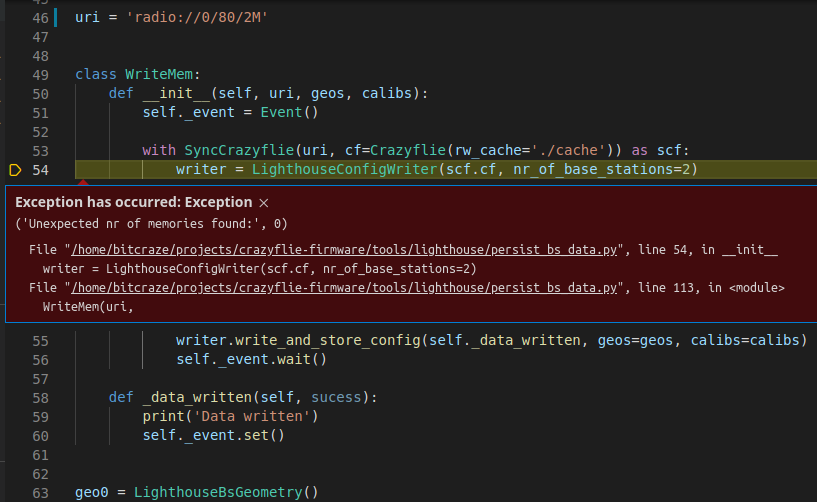
I am a university student trying to set up a lighthouse system for research, and I have been having some issues getting everything set up.
I have gotten the calibration data for two V2 lighthouse base stations manually, but I have been having issues getting this data to tge crazyflie so I can also get the geometry data. Whether I use the write_lighthouse_mem.py or persist_bs_data.py file, the error I am having is identical. In this case, I am using the persist file:
I do not know what this exception means or how to fix it, but I have the newest version of the virtual machine installed, have flashed the crazyflie with the newest version of the firmware, have enabled lighthouse support by creating the config.mk file under this exact file directory: /home/bitcraze/Desktop/projects/crazyflie-firmware/tools/make/
I have been using these two set of instructions:
https://github.com/bitcraze/crazyflie-firmware/blob/2021.01/docs/functional-areas/lighthouse/setting_up.md
https://www.bitcraze.io/documentation/tutorials/getting-started-with-lighthouse/
If someone could tell me how to get the calibration data onto the ram of the crazyflie so I can MANUALLY get the geometry data, that would be greatly appreciated.
Thanks,
Collin R
Beta Was this translation helpful? Give feedback.
All reactions