-
Notifications
You must be signed in to change notification settings - Fork 3
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
main cli done; add todo's; fix getOutArtifact
- Loading branch information
Showing
13 changed files
with
145 additions
and
115 deletions.
There are no files selected for viewing
90 changes: 37 additions & 53 deletions
90
cli/src/main/java/com/github/clagomess/pirilampo/cli/Main.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,72 +1,56 @@ | ||
package com.github.clagomess.pirilampo.cli; | ||
|
||
import com.github.clagomess.pirilampo.core.compilers.FeatureToHTMLCompiler; | ||
import com.github.clagomess.pirilampo.core.compilers.FeatureToPDFCompiler; | ||
import com.github.clagomess.pirilampo.core.compilers.FolderToHTMLCompiler; | ||
import com.github.clagomess.pirilampo.core.compilers.FolderToPDFCompiler; | ||
import com.github.clagomess.pirilampo.core.dto.ParametersDto; | ||
import lombok.extern.slf4j.Slf4j; | ||
|
||
import java.util.Arrays; | ||
|
||
import static com.github.clagomess.pirilampo.core.enums.CompilationArtifactEnum.HTML; | ||
import static com.github.clagomess.pirilampo.core.enums.CompilationArtifactEnum.PDF; | ||
import static com.github.clagomess.pirilampo.core.enums.CompilationTypeEnum.*; | ||
|
||
@Slf4j | ||
public class Main { | ||
/* | ||
public static void main(String[] args) throws Exception { | ||
Main main = new Main(); | ||
log.info("Pirilampo - Ver.: {}", main.getVersion()); | ||
private static final MainOptions mainOptions = new MainOptions(); | ||
|
||
if(args.length > 0){ | ||
CommandLine cmd = consoleOptions(args); | ||
Compilador compilador = new Compilador(); | ||
public static void main(String[] args) { | ||
log.info("Pirilampo - Ver.: {}", Main.class.getPackage().getImplementationVersion()); | ||
|
||
if(cmd.getOptionValue("feature") == null && cmd.getOptionValue("feature_path") == null){ | ||
log.warn("É necessário informar {feature} ou {feature_path}"); | ||
System.exit(1); | ||
} | ||
try { | ||
ParametersDto parameters = mainOptions.getArgs(args); | ||
|
||
if(cmd.getOptionValue("feature") != null){ | ||
compilador.compilarFeature(new Parametro(cmd)); | ||
System.exit(0); | ||
if (parameters.getCompilationType() == FEATURE && | ||
parameters.getCompilationArtifact() == HTML | ||
) { | ||
new FeatureToHTMLCompiler(parameters).build(); | ||
} | ||
|
||
if(cmd.getOptionValue("feature_path") != null){ | ||
compilador.compilarPasta(new Parametro(cmd)); | ||
System.exit(0); | ||
if (parameters.getCompilationType() == FEATURE && | ||
parameters.getCompilationArtifact() == PDF | ||
) { | ||
new FeatureToPDFCompiler(parameters).build(); | ||
} | ||
}else{ | ||
MainUi.launch(MainUi.class); | ||
} | ||
} | ||
private static CommandLine consoleOptions(String[] args){ | ||
Options options = new Options(); | ||
Option option; | ||
options.addOption(new Option("feature", true, "Arquivo *.feature")); | ||
options.addOption(new Option("feature_path", true, "Diretório contendo arquivos *.feature")); | ||
options.addOption(new Option("feature_path_master", true, "Diretório contendo arquivos *.feature master")); | ||
options.addOption(new Option("output", true, "Diretório de saída")); | ||
|
||
option = new Option("name", true, "Nome do projeto"); | ||
option.setRequired(true); | ||
options.addOption(option); | ||
option = new Option("version", true, "Versão"); | ||
option.setRequired(true); | ||
options.addOption(option); | ||
CommandLineParser parser = new DefaultParser(); | ||
HelpFormatter formatter = new HelpFormatter(); | ||
CommandLine cmd = null; | ||
if (Arrays.asList(FOLDER, FOLDER_DIFF).contains(parameters.getCompilationType()) && | ||
parameters.getCompilationArtifact() == HTML | ||
) { | ||
new FolderToHTMLCompiler(parameters).build(); | ||
} | ||
|
||
try { | ||
cmd = parser.parse(options, args); | ||
} catch (ParseException e) { | ||
log.info(e.getMessage()); | ||
formatter.printHelp("Pirilampo", options); | ||
if (parameters.getCompilationType() == FOLDER && | ||
parameters.getCompilationArtifact() == PDF | ||
) { | ||
new FolderToPDFCompiler(parameters).build(); | ||
} | ||
|
||
System.exit(0); | ||
} catch (Throwable e) { | ||
log.error(log.getName(), e); | ||
System.exit(1); | ||
} | ||
return cmd; | ||
} | ||
private synchronized String getVersion(){ | ||
return getClass().getPackage().getImplementationVersion(); | ||
} | ||
*/ | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
91 changes: 37 additions & 54 deletions
91
cli/src/test/java/com/github/clagomess/pirilampo/cli/MainTest.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,69 +1,52 @@ | ||
package com.github.clagomess.pirilampo.cli; | ||
|
||
import com.github.clagomess.pirilampo.cli.Main; | ||
import com.ginsberg.junit.exit.ExpectSystemExitWithStatus; | ||
import lombok.extern.slf4j.Slf4j; | ||
import org.junit.jupiter.api.Test; | ||
import org.junit.jupiter.params.ParameterizedTest; | ||
import org.junit.jupiter.params.provider.CsvSource; | ||
|
||
import java.io.File; | ||
import java.util.Calendar; | ||
import java.util.LinkedList; | ||
import java.util.List; | ||
|
||
@Slf4j | ||
public class MainTest { | ||
/* | ||
@Rule | ||
public final ExpectedSystemExit exit = ExpectedSystemExit.none(); | ||
private File criarPasta(){ | ||
String dir = System.getProperty("java.io.tmpdir"); | ||
dir += File.separator; | ||
dir += "pirilampo_test"; | ||
File f = new File(dir); | ||
if (f.isDirectory() || f.mkdir()) { | ||
dir += File.separator; | ||
dir += (new Long(Calendar.getInstance().getTime().getTime())).toString(); | ||
f = new File(dir); | ||
if(f.mkdir()){ | ||
pastas.add(f); | ||
} | ||
@Test | ||
@ExpectSystemExitWithStatus(1) | ||
public void main_options_validate(){ | ||
Main.main(new String[]{"-projectSource", "aaa"}); | ||
} | ||
|
||
log.info("Pasta de teste: {}", f.getAbsolutePath()); | ||
@ParameterizedTest | ||
@CsvSource(value = { | ||
"FEATURE,HTML,feature/xxx.Feature,", | ||
"FEATURE,PDF,feature/xxx.Feature,", | ||
"FOLDER,HTML,feature,", | ||
"FOLDER_DIFF,HTML,feature,master", | ||
"FOLDER,PDF,feature,", | ||
}) | ||
@ExpectSystemExitWithStatus(0) | ||
public void main_ok( | ||
String compilationType, | ||
String compilationArtifact, | ||
String projectSource, | ||
String projectMasterSource | ||
){ | ||
List<String> argv = new LinkedList<>(); | ||
argv.add("-projectSource"); | ||
argv.add(getClass().getResource(projectSource).getFile()); | ||
|
||
if(projectMasterSource != null) { | ||
argv.add("-projectMasterSource"); | ||
argv.add(getClass().getResource(projectMasterSource).getFile()); | ||
} | ||
|
||
return f; | ||
} | ||
@Test | ||
public void testMain() throws Exception { | ||
exit.expectSystemExit(); | ||
argv.add("-compilationType"); | ||
argv.add(compilationType); | ||
|
||
String outDir = criarPasta().getAbsolutePath(); | ||
Main.main(new String[]{ | ||
"-feature_path", | ||
resourcePath + File.separator + "feature", | ||
"-name", | ||
"XXX", | ||
"-version", | ||
"1.2.3", | ||
"-output", | ||
outDir, | ||
}); | ||
Assert.assertTrue((new File(outDir + File.separator + "index.html")).isFile()); | ||
argv.add("-compilationArtifact"); | ||
argv.add(compilationArtifact); | ||
|
||
outDir = criarPasta().getAbsolutePath(); | ||
Main.main(new String[]{ | ||
"-feature", | ||
resourcePath + File.separator + "feature/xxx.Feature", | ||
"-name", | ||
"XXX", | ||
"-version", | ||
"1.2.3", | ||
"-output", | ||
criarPasta().getAbsolutePath(), | ||
}); | ||
Assert.assertTrue((new File(outDir + File.separator + "xxx.html")).isFile()); | ||
Main.main(argv.toArray(new String[0])); | ||
} | ||
*/ | ||
} |
1 change: 1 addition & 0 deletions
1
cli/src/test/resources/com/github/clagomess/pirilampo/cli/feature/html_embed.html
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1 @@ | ||
<strong>html_embed_txt</strong> |
Binary file added
BIN
+67 Bytes
cli/src/test/resources/com/github/clagomess/pirilampo/cli/feature/smallest.png
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
27 changes: 27 additions & 0 deletions
27
cli/src/test/resources/com/github/clagomess/pirilampo/cli/feature/xxx.Feature
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,27 @@ | ||
# language: pt | ||
# encoding: utf-8 | ||
Funcionalidade: XX | ||
|
||
XXX | ||
|
||
Contexto: XXX | ||
|
||
Dado XXX | ||
E Teste | ||
| Ibagem | | ||
|  | | ||
| <img src="xxx.png"> | | ||
| <img src="xxx.png" width="50"> | | ||
| 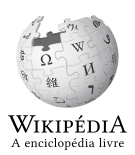 | | ||
| Link Html Embeded: [Link Embeded](html_embed.html) | | ||
| Link Google: [Google](https://www.google.com.br) | | ||
| <strike>strike</strike> | | ||
| <strike>strike<br>strike</strike> | | ||
|
||
|
||
Esquema do Cenário: JJJ | ||
Quando xxx | ||
E YYY | ||
Exemplos: | ||
| a | b | | ||
| c | d | |
Binary file added
BIN
+796 Bytes
cli/src/test/resources/com/github/clagomess/pirilampo/cli/feature/xxx.png
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
28 changes: 28 additions & 0 deletions
28
cli/src/test/resources/com/github/clagomess/pirilampo/cli/master/xxx.Feature
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,28 @@ | ||
# language: pt | ||
# encoding: utf-8 | ||
Funcionalidade: XX | ||
|
||
XXX | ||
|
||
Contexto: XXX | ||
- YYY_MASTER_YYY | ||
|
||
Dado XXX | ||
E Teste | ||
| Ibagem | | ||
|  | | ||
| <img src="xxx.png"> | | ||
| <img src="xxx.png" width="50"> | | ||
| 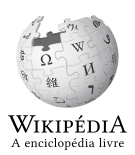 | | ||
| Link Html Embeded: [Link Embeded](html_embed.html) | | ||
| Link Google: [Google](https://www.google.com.br) | | ||
| <strike>strike</strike> | | ||
| <strike>strike<br>strike</strike> | | ||
|
||
|
||
Esquema do Cenário: JJJ | ||
Quando xxx | ||
E YYY | ||
Exemplos: | ||
| a | b | | ||
| c | d | |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -62,5 +62,6 @@ public void build() throws Exception { | |
} | ||
|
||
// @TODO: remove buffer file | ||
// @TODO: add done and took | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters